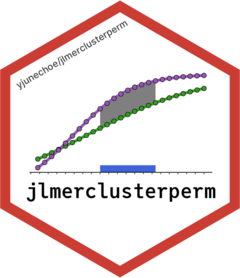
Permute data while respecting grouping structure(s) of observations
Source:R/permute.R
permute_by_predictor.Rd
Permute data while respecting grouping structure(s) of observations
Usage
permute_by_predictor(
jlmer_spec,
predictors,
predictor_type = c("guess", "between_participant", "within_participant"),
n = 1L
)
Arguments
- jlmer_spec
Data prepped for jlmer from
make_jlmer_spec()
- predictors
A vector of terms from the model. If multiple, the must form the levels of one predictor.
- predictor_type
Whether the predictor is
"between_participant"
or"within_participant"
. Defaults to"guess"
.- n
Number of permuted samples of the data to generate. Defaults to
1L
.
Examples
# \donttest{
# Example data setup
chickweights_df <- ChickWeight
chickweights_df <- chickweights_df[chickweights_df$Time <= 20, ]
chickweights_df$DietInt <- as.integer(chickweights_df$Diet)
head(chickweights_df)
#> Grouped Data: weight ~ Time | Chick
#> weight Time Chick Diet DietInt
#> 1 42 0 1 1 1
#> 2 51 2 1 1 1
#> 3 59 4 1 1 1
#> 4 64 6 1 1 1
#> 5 76 8 1 1 1
#> 6 93 10 1 1 1
# Example 1: Spec object using the continuous `DietInt` predictor
chickweights_spec1 <- make_jlmer_spec(
formula = weight ~ 1 + DietInt,
data = chickweights_df,
subject = "Chick", time = "Time"
)
chickweights_spec1
#> ── jlmer specification ───────────────────────────────────────── <jlmer_spec> ──
#> Formula: weight ~ 1 + DietInt
#> Predictors:
#> DietInt: DietInt
#> Groupings:
#> Subject: Chick
#> Trial:
#> Time: Time
#> Data:
#> # A tibble: 533 × 4
#> weight DietInt Chick Time
#> <dbl> <dbl> <ord> <dbl>
#> 1 42 1 1 0
#> 2 51 1 1 2
#> 3 59 1 1 4
#> # ℹ 530 more rows
#> ────────────────────────────────────────────────────────────────────────────────
# Shuffling `DietInt` values guesses `predictor_type = "between_participant"`
reset_rng_state()
spec1_perm1 <- permute_by_predictor(chickweights_spec1, predictors = "DietInt")
#> ℹ Guessed `predictor_type` to be "between_participant"
# This calls the same shuffling algorithm for CPA in Julia, so counter is incremented
get_rng_state()
#> [1] 38
# Shuffling under shared RNG state reproduces the same permutation of the data
reset_rng_state()
spec1_perm2 <- permute_by_predictor(chickweights_spec1, predictors = "DietInt")
#> ℹ Guessed `predictor_type` to be "between_participant"
identical(spec1_perm1, spec1_perm2)
#> [1] TRUE
# Example 2: Spec object using the multilevel `Diet` predictor
chickweights_spec2 <- make_jlmer_spec(
formula = weight ~ 1 + Diet,
data = chickweights_df,
subject = "Chick", time = "Time"
)
chickweights_spec2
#> ── jlmer specification ───────────────────────────────────────── <jlmer_spec> ──
#> Formula: weight ~ 1 + Diet2 + Diet3 + Diet4
#> Predictors:
#> Diet: Diet2, Diet3, Diet4
#> Groupings:
#> Subject: Chick
#> Trial:
#> Time: Time
#> Data:
#> # A tibble: 533 × 6
#> weight Diet2 Diet3 Diet4 Chick Time
#> <dbl> <dbl> <dbl> <dbl> <ord> <dbl>
#> 1 42 0 0 0 1 0
#> 2 51 0 0 0 1 2
#> 3 59 0 0 0 1 4
#> # ℹ 530 more rows
#> ────────────────────────────────────────────────────────────────────────────────
# Levels of a category are automatically shuffled together
reset_rng_state()
spec2_perm1 <- permute_by_predictor(chickweights_spec2, predictors = "Diet2")
#> ℹ Guessed `predictor_type` to be "between_participant"
#> ℹ Shuffling all levels of the factor together ("Diet2", "Diet3", and "Diet4")
reset_rng_state()
spec2_perm2 <- permute_by_predictor(chickweights_spec2, predictors = c("Diet2", "Diet3", "Diet4"))
#> ℹ Guessed `predictor_type` to be "between_participant"
identical(spec2_perm1, spec2_perm2)
#> [1] TRUE
# }