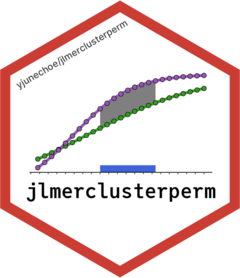
Create a specifications object for fitting regression models in Julia
Source:R/jlmer_spec.R
make_jlmer_spec.Rd
Create a specifications object for fitting regression models in Julia
Usage
make_jlmer_spec(
formula,
data,
subject = NULL,
trial = NULL,
time = NULL,
drop_terms = NULL,
...
)
Arguments
- formula
Model formula in R syntax
- data
A data frame
- subject
Column for subjects in the data.
- trial
Column for trials in the data. Must uniquely identify a time series within subject (for example, the column for items in a counterbalanced design where each subject sees exactly one item).
- time
Column for time in the data.
- drop_terms
(Optional) any terms to drop from the reconstructed model formula
- ...
Unused, for extensibility.
Examples
# Bare specification object (minimal spec for fitting a global model)
spec <- make_jlmer_spec(weight ~ 1 + Diet, ChickWeight)
spec
#> ── jlmer specification ───────────────────────────────────────── <jlmer_spec> ──
#> Formula: weight ~ 1 + Diet2 + Diet3 + Diet4
#> Predictors:
#> Diet: Diet2, Diet3, Diet4
#> Data:
#> # A tibble: 578 × 4
#> weight Diet2 Diet3 Diet4
#> <dbl> <dbl> <dbl> <dbl>
#> 1 42 0 0 0
#> 2 51 0 0 0
#> 3 59 0 0 0
#> # ℹ 575 more rows
#> ────────────────────────────────────────────────────────────────────────────────
# Constraints on specification for CPA:
# 1) The combination of `subject`, `trial`, and `time` must uniquely identify rows in the data
# 2) `time` must have constant sampling rate (i.e., evenly spaced values)
spec_wrong <- make_jlmer_spec(
weight ~ 1 + Diet, ChickWeight,
time = "Time"
)
#> ! Grouping column "Time" does not uniquely identify rows in the data.
#> ! Sampling rate for the `time` column "Time" is not constant - may affect interpretability of results.
unique(ChickWeight$Time)
#> [1] 0 2 4 6 8 10 12 14 16 18 20 21
# Corrected specification for the above
spec_correct <- make_jlmer_spec(
weight ~ 1 + Diet, subset(ChickWeight, Time <= 20),
subject = "Chick", time = "Time"
)
spec_correct
#> ── jlmer specification ───────────────────────────────────────── <jlmer_spec> ──
#> Formula: weight ~ 1 + Diet2 + Diet3 + Diet4
#> Predictors:
#> Diet: Diet2, Diet3, Diet4
#> Groupings:
#> Subject: Chick
#> Trial:
#> Time: Time
#> Data:
#> # A tibble: 533 × 6
#> weight Diet2 Diet3 Diet4 Chick Time
#> <dbl> <dbl> <dbl> <dbl> <ord> <dbl>
#> 1 42 0 0 0 1 0
#> 2 51 0 0 0 1 2
#> 3 59 0 0 0 1 4
#> # ℹ 530 more rows
#> ────────────────────────────────────────────────────────────────────────────────